The cool thing about templates is that it lets you easily structure your content display without all the hassle of string concatenation. For example, say I had a data set containing an ID and a name and I wanted to display the name as a hyperlink.
I could do something like this:
var clRec = "" ; for ( var i=0; i<client.name.length; i++) { clRec += "<li><a href='clients/" +client.id[i]+ "'>" + client.name[i] + "</a></li>" ; } |
but to me, this seems much more readable and maintainable:
<li><a href= "clients/${id}" >${name}</a></li> |
The second code snippet is a template I’ve created to render a hyperlinked name in a list and it’s very easy to do. First, include both jQuery and the jQuery Template plugin into your page:
<script src= "jquery.tmpl.js" type= "text/javascript" ></script> |
Next, define the data that you’d like to see rendered. This could be data derived from an XHR call or something you’ve predefined, like this:
var clientData = [ { name: "Rey Bango" , id: 1 }, { name: "Mark Goldberg" , id: 2 }, { name: "Jen Statford" , id: 3 } ]; |
Now, define your template:
<script id= "clientTemplate" type= "text/html" > <li><a href= "clients/${id}" >${name}</a></li> </script> |
Yep, the “script” tags are necessary and used because they allow your template to be embedded within the body of the page. Notice that we’ve defined the placeholders as ${id} & ${name}, the same names used in the identifiers in our data. The “${}” tells the template parser that the field needs to be replaced with the expected value.
Then call the jQuery Template plugin to render the data using your template:
$( "#clientTemplate" ).tmpl(clientData).appendTo( "ul" ); |
The plugin’s .tmpl() method accepts the data we’ve defined and handles the parsing of the template we’ve selected. Then we use jQuery’s .appendTo() method to append the results to an unordered list tag. This gives me the following results:
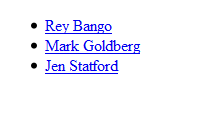
That’s it! Told you it was simple. 

I’ll go into more advanced details during my presentation but this should give you something to start with in the meantime. Here’s the full source for you to use:
<!DOCTYPE html> < html lang = "en" > < head > < meta http-equiv = "Content-Type" content = "text/html; charset=utf-8" /> < meta name = "robots" content = "noindex" /> < title >Template Test</ title > < script src = "jquery.tmpl.js" type = "text/javascript" ></ script > < script type = "text/javascript" > $(document).ready(function() { var clientData = [ { name: "Rey Bango", id: 1 }, { name: "Mark Goldberg", id: 2 }, { name: "Jen Statford", id: 3 } ]; $("#clientTemplate").tmpl(clientData).appendTo( "ul" ); }); </ script > < script id = "clientTemplate" type = "text/html" > < li >< a href = "clients/${id}" >${name}</ a ></ li > </ script > </ head > < body > < ul ></ ul > </ body > </ html > |
No comments:
Post a Comment