Introduction
The SAP .Net Connector is a software component supplied by SAP that makes it possible to communicate between an SAP host and a .Net program. Any .Net language can be used with the SAP .Net Connector.
Where to Find It
You can download the SAP .Net Connector from http://service.sap.com/connectors. You will have to provide your SAP Marketplace user ID and password.
What it Does
The SAP .Net Connector gives a .Net program access to an SAP host. The .Net program can logon to an SAP host and issue SAP Remote Function Calls. Parameters can be passed in to an RFC, and results data can be passed back to the .Net program.
A .Net program can also establish a connection to an SAP IDOC Gateway. The .Net program uses the SAP .Net Connector to send and receive IDOCs. There is full support for transactional integrity for IDOCS moving back and forth between the SAP host and the .Net program.
With this functionality, a Microsoft .Net program has all that it needs to query SAP metadata, call ABAP functions, or send and receive IDOCs.
What it Doesn’t Do
While the SAP .Net Connector takes care of most of the heavy lifting in communicating with an SAP host, there is still considerable work to be done before you can get something useful from it.
In particular, there is no functionality built-in to the SAP .Net Connector for parsing the various data fields from an IDOC. It is up to the receiving program to know what the format of the IDOC is, and how to extract the data from the IDOC.
One other issue to deal with is that the SAP .Net Connector is built for Visual Studio 2003. There is a work around that is easy to use that enables you to use the SAP .Net Connector with newer versions of Visual Studio.
--------------------------------------------------------------------------------------------------------------
To illustrate the process, we will build up, over the course of several blog posts, a system that is capable of receiving an IDOC from an SAP host. This system will be known as the Three Letter Acronym system, or TLA.
The approach is to first add the SAP .Net Connector to a Visual Studio 2003 project. Then, we will attach to an SAP host to browse available functions. We will select the desired functions to be included in our SAP proxy.
Then, we will build the VS 2003 project into a DLL that can be used in Visual Studio 2008.
We don’t need the default Class1.cs file that is created, so close the Class1.cs module that is displayed, and remove Class1.cs from the TlaSapProxy project.
For Destination Type, select Custom Logon Settings from the drop down list. Enter a valid SAP Username, Client, and Password. For the AppServerHost, enter the SAP host name, or the IP address of the desired SAP host. Be sure to fill in the Language field.
If things go well, at this point you will have an SAP Application Server established. You should see two new nodes on the Server Explorer display: BOR and Functions. Under BOR, you can expand either the Alphabetical or Hierarchical nodes to see available object definitions.
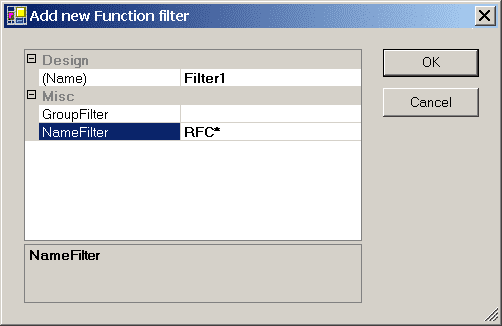
For our TLA SAP Proxy we are building, we only need RFC_GET_SYSTEM_INFO. As with most SAP functions, RFC_GET_SYSTEM_INFO returns an object of a custom type that includes all of the results of the function call.
From the Visual Studio 2003 Solution Explorer, right click on the TlaSapProxy project and choose Add, then Add New Item. Scroll through the list of Templates and click on SAP Connector Proxy, then click OK to add the new item to the project.
What you have at that point is a Visual Studio design surface to which we can add SAP function definitions. The design surface is displayed as SAPProxy1.sapwsdl. We are basically creating a WSDL file that will be used to generate our desired DLL.
To include an SAP function, in our case RFC_GET_SYSTEM_INFO, display the Server Explorer. Expand the SAP Application Servers to the desired server, then click on RFC_GET_SYSTEM_INFO. Drag RFC_GET_SYSTEM_INFO to the SAPProxy1.sapwsdl and drop it there.
The screen will display ‘Retrieve Data.’ When it is done, you will see two things added to the WSDL. First is the function call definition (method). Second is a class definition for what is returned from the function. You can right click on these and select Properties to view details of what is contained therein.
Because we selected a Class Library as our starting project template, our project is already set to build a class module (DLL). To build the DLL, right click on the TlaSapProxy project and select Build.
This will compile our WSDL file into a DLL file that we will be able to use in Visual Studio 2008.
We do this by establishing a connection to an SAP host, selecting the desired functions, and creating an SAP proxy object that contains the desired functions. When we build this project, the resulting DLL can be used in Visual Studio 2008.
--------------------------------------------------------------------------------------------------------------
We will reference that DLL in a Visual Studio 2008, and use it to retrieve system information from an SAP host.
As a demonstration, we are creating the TLA System, which exists solely to demonstrate certain SAP .Net Connector programming techniques.
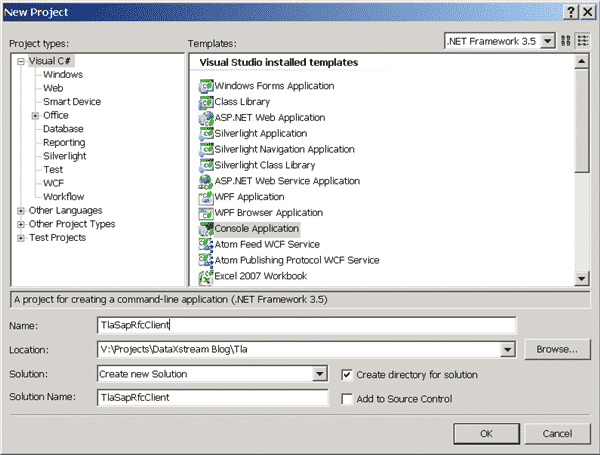
In the Solution Explorer, right click References and choose Add Reference. Using the Browse tab, find the TlaSapProxy.dll file from the TlaSapProxy project. Typically, this will be in the bin\debug folder of the TlaSapProxy folder. Click the OK button to add the reference to your project.
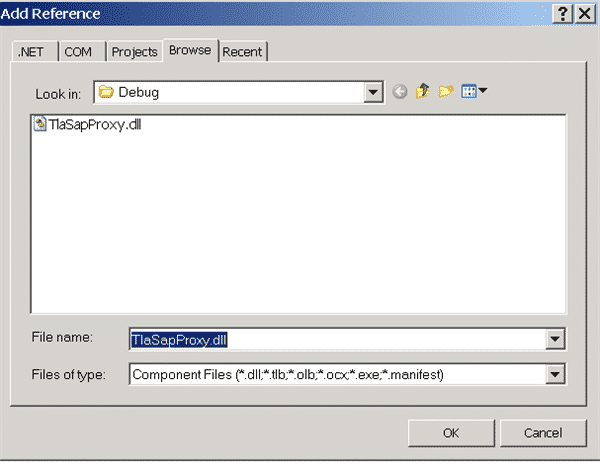
When we create an instance of the proxy in line 11, we pass in an SAP connection string. We create an object of type SAPProxy1 because that is what we named it in our Visual Studio 2003 project.
In line 20, we create an object of type RFCSI, because that is what our RFC call to Rfc_Get_System_Info will return. The type definition for RFCSI was also included in the proxy.
In line 22 we make the RFC call to the SAP host. We get full IntelliSense support that describes the parameters required.
Here we have described how to use that DLL in a Visual Studio 2008 project.
The SAP .Net Connector is a software component supplied by SAP that makes it possible to communicate between an SAP host and a .Net program. Any .Net language can be used with the SAP .Net Connector.
Where to Find It
You can download the SAP .Net Connector from http://service.sap.com/connectors. You will have to provide your SAP Marketplace user ID and password.
What it Does
The SAP .Net Connector gives a .Net program access to an SAP host. The .Net program can logon to an SAP host and issue SAP Remote Function Calls. Parameters can be passed in to an RFC, and results data can be passed back to the .Net program.
A .Net program can also establish a connection to an SAP IDOC Gateway. The .Net program uses the SAP .Net Connector to send and receive IDOCs. There is full support for transactional integrity for IDOCS moving back and forth between the SAP host and the .Net program.
With this functionality, a Microsoft .Net program has all that it needs to query SAP metadata, call ABAP functions, or send and receive IDOCs.
What it Doesn’t Do
While the SAP .Net Connector takes care of most of the heavy lifting in communicating with an SAP host, there is still considerable work to be done before you can get something useful from it.
In particular, there is no functionality built-in to the SAP .Net Connector for parsing the various data fields from an IDOC. It is up to the receiving program to know what the format of the IDOC is, and how to extract the data from the IDOC.
One other issue to deal with is that the SAP .Net Connector is built for Visual Studio 2003. There is a work around that is easy to use that enables you to use the SAP .Net Connector with newer versions of Visual Studio.
--------------------------------------------------------------------------------------------------------------
Introduction
We will use Visual Studio 2003 and create a Dynamic Link Library (DLL) that encapsulates the desired SAP .Net Connector functionality. We can use the DLL that we create in a Visual Studio 2008 project.To illustrate the process, we will build up, over the course of several blog posts, a system that is capable of receiving an IDOC from an SAP host. This system will be known as the Three Letter Acronym system, or TLA.
Build an SAP Proxy
Our first task is to use the SAP .Net Connector to build a proxy that we can incorporate into Visual Studio 2008 projects.The approach is to first add the SAP .Net Connector to a Visual Studio 2003 project. Then, we will attach to an SAP host to browse available functions. We will select the desired functions to be included in our SAP proxy.
Then, we will build the VS 2003 project into a DLL that can be used in Visual Studio 2008.
Start a Visual Studio 2003 Project
After you have installed the SAP .Net Connector, start a new Visual Studio for .Net 2003 project. Name the project “TlaSapProxy.” Choose the “Class Library” C# template.
Creating a new SAP Proxy project
Add a Reference to the SAP .Net Connector
Now we need to add a reference to the SAP .Net Connector. From the Solution Explorer view, expand TlaSapProxy / References. Right click on References and choose Add Reference. From the .Net tab, double-click both SAP.Connector.dll and SAP.Connector.Rfc. Click OK to add the reference to your project.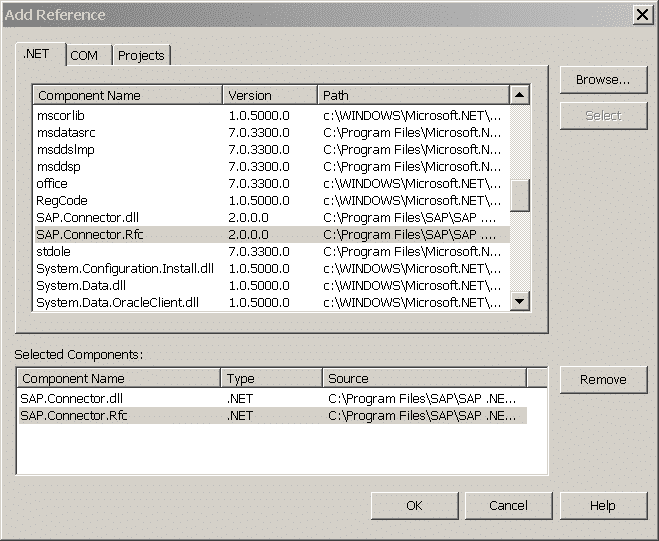
Adding References to SAP .Net Connector
Establish a Connection to an SAP Host
We will use the Visual Studio design time environment to attach to an SAP Host. From the Visual Studio View menu, select Server Explorer. Expand the SAP node. Right click on Application Servers and select Add Application Server.For Destination Type, select Custom Logon Settings from the drop down list. Enter a valid SAP Username, Client, and Password. For the AppServerHost, enter the SAP host name, or the IP address of the desired SAP host. Be sure to fill in the Language field.
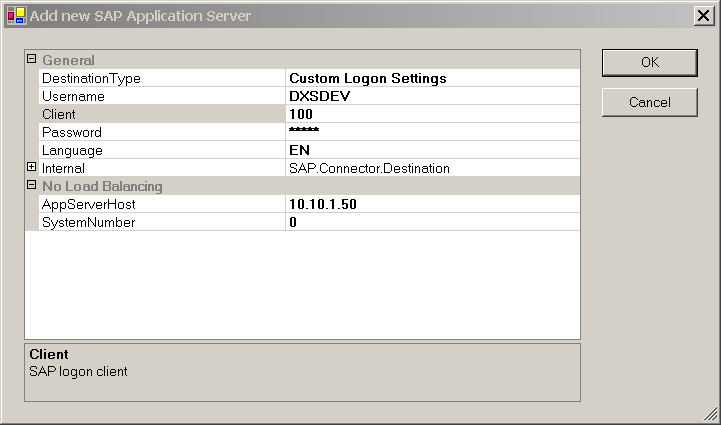
SAP Server Connection Properties
Browse SAP Functions
To display an SAP function using the Server Explorer, you have to create a function ‘filter,’ which selects which functions from the SAP Host you want to display. To do this, right click on Functions and choose Add Function Filter. In our proxy, we are going to be using some RFC functions, so, in the NameFilter field, enter RFC* (the asterisk is a wild card). Click OK, then expand Functions, then expand Filter1. After a moment, the screen will display a list of SAP functions that match our filter.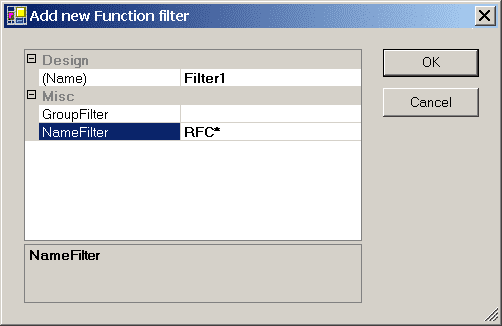
Creating a New Function Filter
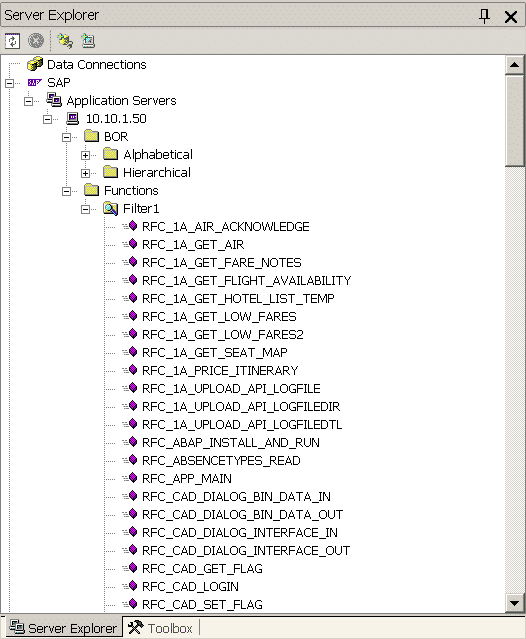
SAP Function Filter Display
Select Desired SAP Functions
As you can see, there are many functions to choose from. When we build our SAP proxy, we will select just the functions we need.For our TLA SAP Proxy we are building, we only need RFC_GET_SYSTEM_INFO. As with most SAP functions, RFC_GET_SYSTEM_INFO returns an object of a custom type that includes all of the results of the function call.
From the Visual Studio 2003 Solution Explorer, right click on the TlaSapProxy project and choose Add, then Add New Item. Scroll through the list of Templates and click on SAP Connector Proxy, then click OK to add the new item to the project.
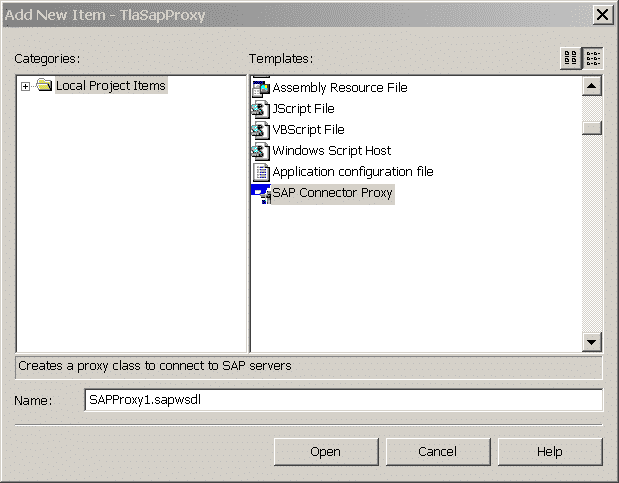
Adding an SAP Connector Proxy Object
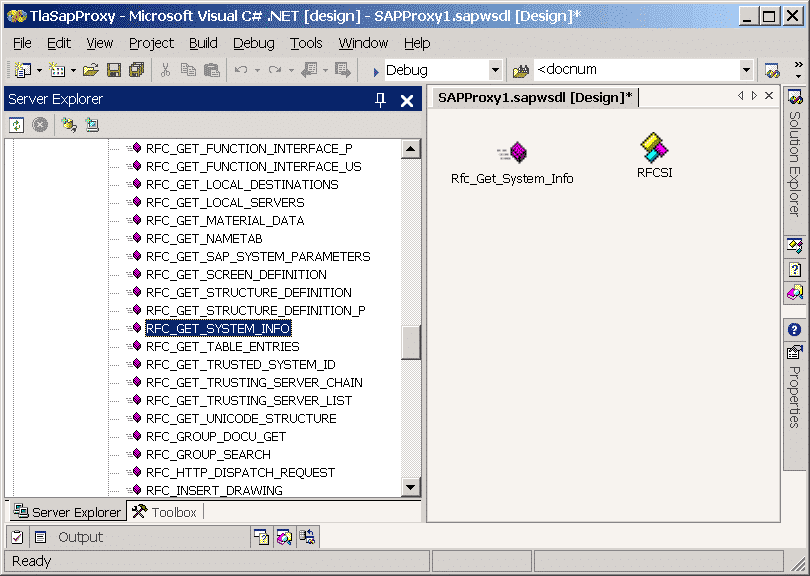
Adding SAP Functions to a Proxy
The screen will display ‘Retrieve Data.’ When it is done, you will see two things added to the WSDL. First is the function call definition (method). Second is a class definition for what is returned from the function. You can right click on these and select Properties to view details of what is contained therein.
Building the Project
Now that we have selected all of the desired SAP functions that we want to include in our proxy, we are ready to build the project.Because we selected a Class Library as our starting project template, our project is already set to build a class module (DLL). To build the DLL, right click on the TlaSapProxy project and select Build.
This will compile our WSDL file into a DLL file that we will be able to use in Visual Studio 2008.
Summary
The SAP .Net Connector is built for Visual Studio 2003. By using Visual Studio 2003 to create a DLL, we can use the SAP .Net Connector in Visual Studio 2008 (or later).We do this by establishing a connection to an SAP host, selecting the desired functions, and creating an SAP proxy object that contains the desired functions. When we build this project, the resulting DLL can be used in Visual Studio 2008.
--------------------------------------------------------------------------------------------------------------
Introduction
Our previous post described how to use Visual Studio 2003 and the SAP .Net Connector to create a Dynamic Link Library containing the desired SAP functionality. The DLL contains proxies for each SAP function that we want to utilize.We will reference that DLL in a Visual Studio 2008, and use it to retrieve system information from an SAP host.
As a demonstration, we are creating the TLA System, which exists solely to demonstrate certain SAP .Net Connector programming techniques.
Using the SAP .Net Connector Proxy
Now we are ready to put our SAP .Net Connector Proxy to work. This will allow us to use the SAP .Net Connector in the modern Visual Studio environment.Start a Visual Studio 2008 Project
Start a new Visual Studio 2008 Project. Use the Console Application template, and name the solution TlaSapRfcClient.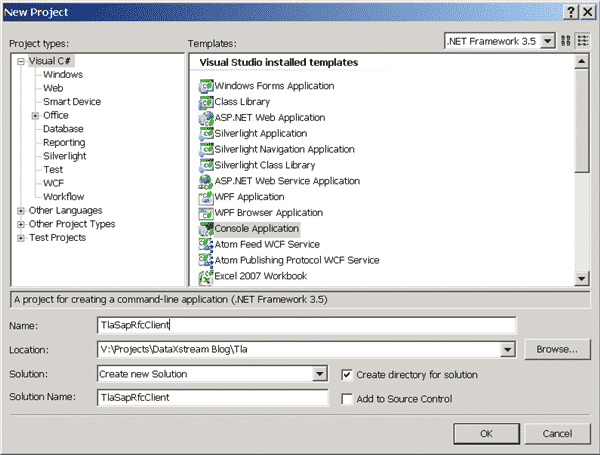
Creating a new console application
Add a Reference to the TLA SAP Proxy
Now we need to add a reference to the DLL we created earlier.In the Solution Explorer, right click References and choose Add Reference. Using the Browse tab, find the TlaSapProxy.dll file from the TlaSapProxy project. Typically, this will be in the bin\debug folder of the TlaSapProxy folder. Click the OK button to add the reference to your project.
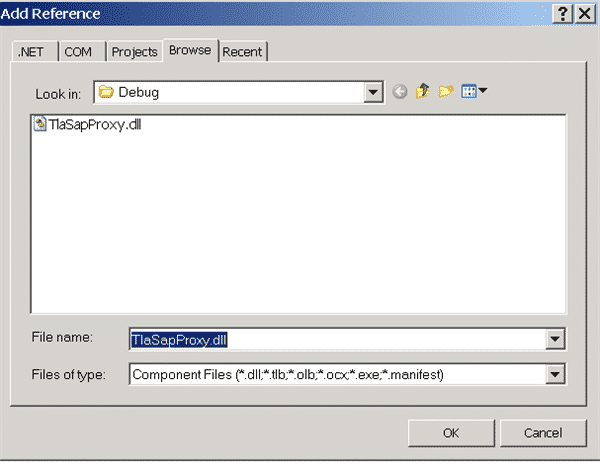
Adding a reference to the proxy
C# Code
Here is a Visual Studio 2008 C# program that uses the SAP .Net Connector proxy that we built earlier using Visual Studio 2003.
C# program listing
In line 20, we create an object of type RFCSI, because that is what our RFC call to Rfc_Get_System_Info will return. The type definition for RFCSI was also included in the proxy.
In line 22 we make the RFC call to the SAP host. We get full IntelliSense support that describes the parameters required.
Summary
The SAP .Net Connector is built for Visual Studio 2003. By using Visual Studio 2003 to create a DLL, we can use the SAP .Net Connector in Visual Studio 2008 (or later).Here we have described how to use that DLL in a Visual Studio 2008 project.
SAP.Net.Connector.dll is enough or do we need to install using SAP.Net:Setup.msi for working with SAP IDocs and sap connection?
ReplyDeleteSAP.Net.Connector.dll is enough.
ReplyDeleteThanks...Patel.
ReplyDeleteThis Post is really helpful and easy to understand.
Hi Patel,
ReplyDeleteIs SAP.Net.Connector.dll and SAP.Net Connector 3.0 (sapnco) are both the same or different version.
Thanks
Any way to extract dll from RFC using c# code ?
ReplyDeleteonline coding courses for kids Wow, cool post. I'd like to write like this too - taking time and real hard work to make a great article... but I put things off too much and never seem to get started. Thanks though.
ReplyDelete