The SAP .Net Connector 3.0 (NCo 3.0) offers many improvements over the 2.0 version of that product. Unfortunately, SAP no longer offers example .NET code. This blog attempts to fill that gap by describing how to build a simple RFC Client using SAP .Net Connector (NCo) 3.0. Click here to request a .zip file containing a copy of the source code.
The sample program displays details about companies defined by SAP. There are two BAPI calls involved, BAPI_COMPANY_GETLIST and BAPI_COMPANY_GETDETAIL.
Along with the SAP .Net Connector 3.0, we are using Microsoft Visual Studio 2010 and the Microsoft .Net Framework 4.0 to build our sample. Prior to starting, you will have to download and install NCo 3.0 (OSS login required).
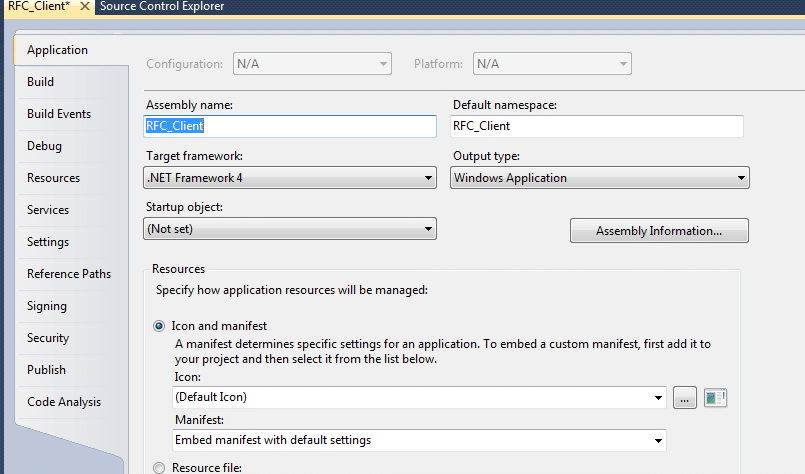
Add references to the SAP .Net Connector 3.0. There are two DLLs, sapnco.dll and sapnco_utils.dll.
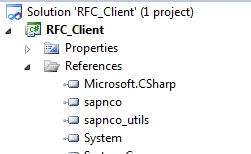

The SAP .Net Connector 3.0 includes sample app.config files. For the RFC client, be sure there is a sectionGroup definition for <ClientSettings>.
The SAP host is defined by the destinations section. Within the destination, the Name field identifies this app.config entry to our program code. Specify the appropriate user name, password, SAP host name, client and system number in the destination section.


The rest of the code is regular Windows Forms programming. Your code will need to wire up the List Box changed events so that the Property Grid will display the details of the selected company.
The sample program displays details about companies defined by SAP. There are two BAPI calls involved, BAPI_COMPANY_GETLIST and BAPI_COMPANY_GETDETAIL.
Along with the SAP .Net Connector 3.0, we are using Microsoft Visual Studio 2010 and the Microsoft .Net Framework 4.0 to build our sample. Prior to starting, you will have to download and install NCo 3.0 (OSS login required).
Setting up the Project
Using Visual Studio 2010, create a new Windows Form project. In the project properties, be sure to set the Target Framework to .Net Framework 4.0.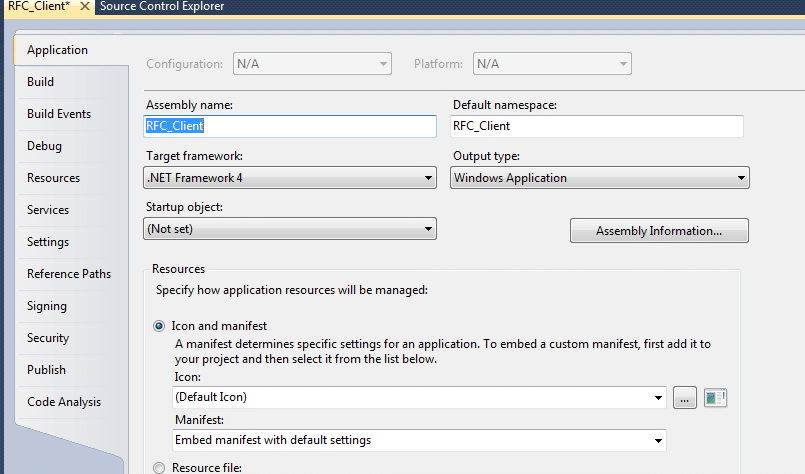
Add references to the SAP .Net Connector 3.0. There are two DLLs, sapnco.dll and sapnco_utils.dll.
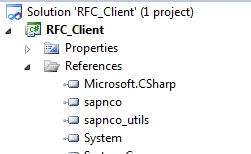
Design the Form
Add two controls to the form. The first is a List Box, which will contain the list of available SAP companies. The second control to add is a Property Grid control, which is used to display the details of a particular SAP company when the user selects one from the List Box.
Using App.Config to define the SAP Connections
There are several methods you can use in your solutions to define a particular SAP host. For this example, we are using an app.config file to define our SAP host.The SAP .Net Connector 3.0 includes sample app.config files. For the RFC client, be sure there is a sectionGroup definition for <ClientSettings>.
The SAP host is defined by the destinations section. Within the destination, the Name field identifies this app.config entry to our program code. Specify the appropriate user name, password, SAP host name, client and system number in the destination section.

The BAPIs
Use the SAP transaction SE37 to determine the parameters for functions BAPI_COMPANY_GETLIST and BAPI_COMPANY_GETDETAIL. This will tell us what parameters need to be passed in and where to find the results that are returned to our code.The Code
During the form load event, we want to populate our List Box with the defined companies from SAP.- Acquire a valid RfcDestination to use. Use method RfcDestinationManager.GetDestination() to do this. GetDestination() requires a parameter to determine which SAP host to refer to. Pass in the Name of the SAP host you previously defined in the app.config file. (Line 60)
- Now that we have a valid RfcDestination object, we need access to the SAP Repository. The repository contains information about the BAPI calls we are going to make. The RfcRepository is an attribute of an RfcDestination object. (Line 62)
- Using the RfcRepository object, acquire a reference to the SAP BAPI by calling method RfcRepository.CreateFunction() method. Pass in the name of the desired function as a string parameter to CreateFunction(). This method returns an object we can use to setup parameters, invoke the function, and retrieve results.
- Now we can make the RFC calls into SAP. Using the IRfcFunction object returned by CreateFunction(), call the Invoke() method, passing in our RfcDestination object as parameter. This will make the RFC call into SAP, and provides the results via our IRfcFunction object. (Line 68)
- SAP function BAPI_GET_COMPANYLIST returns a table of company records. We use an instance of an IRfcTable object that we retrieve by calling the GetTable() method on the IRfcFunction object. To get a desired table, pass in the table name to the GetTable() method. (Line 70)
- We now have an instance of an IRfcTable object that contains company information through which we can iterate. It is possible to iterate through the data by setting the CurrentIndex property on the returned table. For a particular row in the table, it is possible to access values using the GetString() method. (Lines 75-79)
- For each company, get the CompanyNumber, and use that as a parameter to SAP function BAPI_COMPANY_GETDETAIL. BAPI_COMPANY_GETDETAIL returns detailed information about that particular SAP company. The techniques are the same as before, with the additional step of using the SetValue() method on the IRfcFunction object to pass in the company number as a parameter. (Lines 78-84)
- BAPI_COMPANY_GETDETAIL returns data as an instance of the IRfcStructure interface. Access individual field data of the company record, using the GetString() method of the IRfcStructure object instance. (Lines 88-99)
- Extract the company details as desired. Add them to some object you create and then add that object to the List Box control. (Line 101)

The rest of the code is regular Windows Forms programming. Your code will need to wire up the List Box changed events so that the Property Grid will display the details of the selected company.
No comments:
Post a Comment